2D游戏入门——小狐狸系列(七)
作者:互联网
Session07:跳跃动画 LayerMask
添加动画、设置转换条件
这节课我们来为人物添加跳跃动画。
按照上节课的方法,添加jump和fall的动画到Player,然后回到Animator,添加这样的transition关系
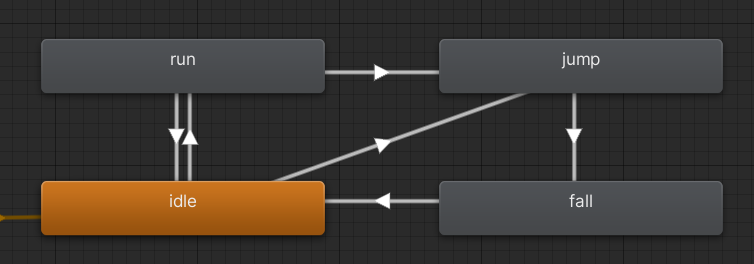
并设置condition,添加两个bool类型的参数,分别对应jumping、falling,处于idle和run的状态时如果jumping为true就切换为jump动画,处于jump状态时如果falling为true并且jumping为false就切换为fall动画,处于fall状态时如果falling为false就切换为idle动画。
写代码
void SwitchAnimation()
{
//正在跳跃
if (animator.GetBool("jumping"))
{
//y轴向上的速度为0,开始下落
if(rb.velocity.y < 0)
{
animator.SetBool("jumping", false);
animator.SetBool("falling", true);
}
}
//正在下落
if (animator.GetBool("falling"))
{
if(rb.velocity.y == 0)
{
animator.SetBool("falling", false);
}
}
}
这样就可以实现基本的跳跃和下落动画了。
但注意到if(rb.velocity.y == 0)
这个判断语句,当人物处于斜坡上时y方向的速度也不为0,可能会一直处于falling状态。
所以这里要用到LayerMask
LayerMask
我们为人物脚本新添加一个组件public LayerMask ground;
因为我们想要检测是否碰撞到地面,所以我们为Tilemap添加一个Layer叫Ground,并为它选择:
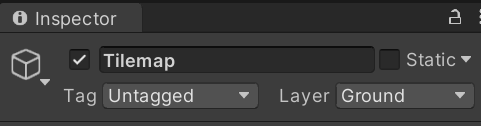
然后回到Player,将刚才声明的LayerMask选择为Ground:
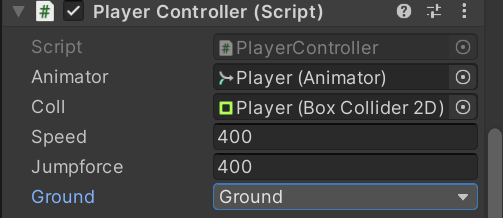
完善代码:
因为要检测碰撞,所以还需要一个collider组件
public class PlayerController : MonoBehaviour
{
public LayerMask ground;
public Collider2D coll;
// Update is called once per frame
void Update()
{
Movement();
SwitchAnimation();
}
void SwitchAnimation()
{
//正在跳跃
if (animator.GetBool("jumping"))
{
//y轴速度消失,开始下落
if(rb.velocity.y < 0)
{
animator.SetBool("jumping", false);
animator.SetBool("falling", true);
}
}
//正在下落
if (animator.GetBool("falling"))
{
if(coll.IsTouchingLayers(ground))
{
animator.SetBool("falling", false);
}
}
}
}
标签:动画,入门,狐狸,2D,jumping,SetBool,falling,LayerMask,animator 来源: https://blog.csdn.net/Ricardo_M_Hu/article/details/121189273